Gale and Shapley proved that there is a stable set of engagements for any set of preferences and the first link above gives their algorithm for finding a set of stable engagements. We're provided with a list of men (M) and women (W), indicating each mans (and womans) spousal preferences ranked from highest to lowest. Nov 04, 2013 Gale-Shapley. Gale-Shapley stable matching algorithm implemented in Java. Input: a text file that defines the preference list of each man and woman, where there are n number of men and n number of women. Each person lists n people in their pref list. Here is the source code of the Java Program to Implement Gale Shapley Algorithm. The Java program is successfully compiled and run on a Windows system. The Program is attached below. Java & Programming Projects for $15. Stable matching gale shapley algorithm, program gale shapley algorithm, mysql implementation man day estimation. Here is the source code of the Java Program to Implement Gale Shapley Algorithm. The Java program is successfully compiled and run on a Windows system. Theses and Dissertations Available from Pro.
The Gale-Shapley Algorithm is an important part of Java. Pseudo code of the Algorithm. Step 1 – Set all m belongs to M and w belongs to W are free or not engaged. While there is a man m who is single and has not selected any woman Step 2- Choose such a man m. The Gale–Shapley algorithm (also known as the Deferred Acceptance algorithm) involves a number of 'rounds' (or ' iterations '): In the first round, first a) each unengaged man proposes to the woman he prefers most, and then b) each woman replies 'maybe' to her suitor she most prefers and 'no' to all other suitors. Following is Gale–Shapley algorithm to find a stable matching: The idea is to iterate through all. Java program for stable marriage problem. Import java.util.

Gale Shapley Java Program Pdf
Gale Shapley Java Programming

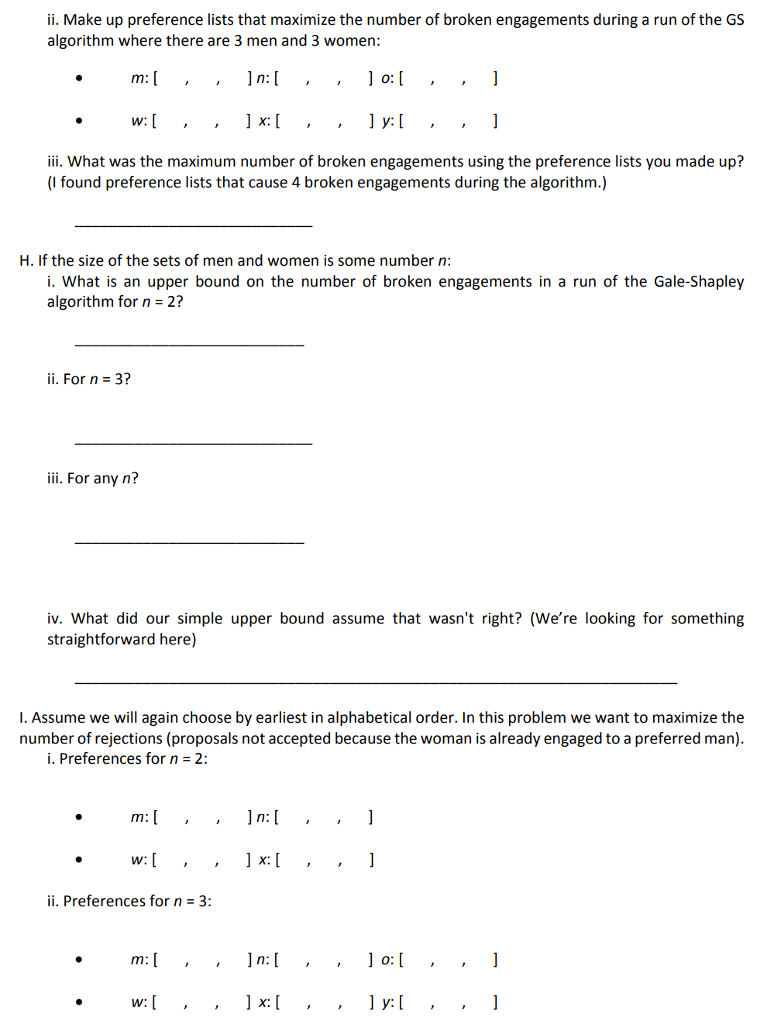
Java - Gale-Shapley Algorithm - Stack Overflow
The Stable Marriage ProblemThe Stable Marriage problem is a classical combinatorial problemthat belongs to the family of stable matching problems. An instanceof the Stable Marriage problem involves n men and n women,and each person ranks all members of the opposite sex in strictorder of preference. Given a matching M of the men andwomen (in other words, a one-one correspondence between them),we say that M admits a blocking pair if there is a manm and a woman w, not matched together in M, such that m prefersw to his partner in M and w prefers m to her partner in M.The existence of a blocking pair (m,w) represents a situation inwhich the man m and woman w involved would prefer to disregardtheir assigned spouses in the matching and have an affair,thereby undermining the integrity of the matching.
Gale Shapley Java Programs
Code:
public class GaleShapley
{
private int N, engagedCount;
private String[][] menPref;
private String[][] womenPref;
private String[] men;
private String[] women;
private String[] womenPartner;
private boolean[] menEngaged;
/** Constructor **/
public GaleShapley(String[] m, String[] w, String[][] mp, String[][] wp)
{
N = mp.length;
engagedCount = 0;
men = m;
women = w;
menPref = mp;
womenPref = wp;
menEngaged = new boolean[N];
womenPartner = new String[N];
calcMatches();
}
/** function to calculate all matches **/
private void calcMatches()
{
while (engagedCount < N)
{
int free;
for (free = 0; free < N; free++)
if (!menEngaged[free])
break;
for (int i = 0; i < N && !menEngaged[free]; i++)
{
int index = womenIndexOf(menPref[free][i]);
if (womenPartner[index] null)
{
womenPartner[index] = men[free];
menEngaged[free] = true;
engagedCount++;
}
else
{
String currentPartner = womenPartner[index];
if (morePreference(currentPartner, men[free], index))
{
womenPartner[index] = men[free];
menEngaged[free] = true;
menEngaged[menIndexOf(currentPartner)] = false;
}
}
}
}
printCouples();
}
/** function to check if women prefers new partner over old assigned partner **/
private boolean morePreference(String curPartner, String newPartner, int index)
{
for (int i = 0; i < N; i++)
{
if (womenPref[index][i].equals(newPartner))
return true;
if (womenPref[index][i].equals(curPartner))
return false;
}
return false;
}
/** get men index **/
private int menIndexOf(String str)
{
for (int i = 0; i < N; i++)
if (men[i].equals(str))
return i;
return -1;
}
/** get women index **/
private int womenIndexOf(String str)
{
for (int i = 0; i < N; i++)
if (women[i].equals(str))
return i;
return -1;
}
/** print couples **/
public void printCouples()
{
System.out.println('Couples are : ');
for (int i = 0; i < N; i++)
{
System.out.println(womenPartner[i] +' '+ women[i]);
}
}
/** main function **/
public static void main(String[] args)
{
System.out.println('Gale Shapley Marriage Algorithmn');
/** list of men **/
String[] m = {'M1', 'M2', 'M3', 'M4', 'M5'};
/** list of women **/
String[] w = {'W1', 'W2', 'W3', 'W4', 'W5'};
/** men preference **/
String[][] mp = {{'W5', 'W2', 'W3', 'W4', 'W1'},
{'W2', 'W5', 'W1', 'W3', 'W4'},
{'W4', 'W3', 'W2', 'W1', 'W5'},
{'W1', 'W2', 'W3', 'W4', 'W5'},
{'W5', 'W2', 'W3', 'W4', 'W1'}};
/** women preference **/
String[][] wp = {{'M5', 'M3', 'M4', 'M1', 'M2'},
{'M1', 'M2', 'M3', 'M5', 'M4'},
{'M4', 'M5', 'M3', 'M2', 'M1'},
{'M5', 'M2', 'M1', 'M4', 'M3'},
{'M2', 'M1', 'M4', 'M3', 'M5'}};
GaleShapley gs = new GaleShapley(m, w, mp, wp);
}
}
Output:
Gale Shapley Marriage Algorithm
Couples are :
M4 W1
M2 W2
M5 W3
M3 W4
M1 W5
More Java Programs: